Physical structure:
An easy to see that the combolist consist of three(body,head,list)main
parts these parts are combine to complete the physical structure of the
combolist control , in this article I will try to explain an easy way how to create combolist control From Scratch , not nice look one only but also
with straight main operations ,methods and properties .
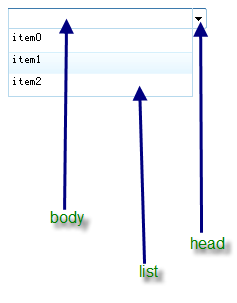
pic1
as you see above the pic1 contain the three parts are
1-body :the plot where the selected item is placed
2-head: the plot that we can control the hiding and show the element list.
3-list: the plot that contain all combolist elements(items)
logical structure of combolist :
we discuss the logical structure from three aspects(css,js,and html)
the css to control the style ( make nice or the dress it up )
the css style is tracing the pic1 three parts.

Code
/*div tag*/
.ComboList
{
width:200px;
height:400px;
margin-left:0px;
margin-top:0px;
font-family: 宋体;
font-size:12px;
color:black;
cursor:default;
}
/*span*/
.ComboList .headPar
{
width:17px;
height:21px;
margin-left:182px;
position:absolute;
background-image:url('images/combolist.png');
background-repeat:no-repeat;
}
/*input tag*/
.ComboList .bodyPart
{
border-top: 1px #3D7BAD solid;
border-bottom: 1px #B7D9ED solid;
border-left:1px #A4C9E3 solid;
border-right:1px #A4C9E3 solid;
height:17px;
position:absolute;
width:181px;
font-family: 宋体;
font-size:12px;
color:black;
}
/*ul*/
.ComboList .ListPart
{
margin-top:21px;
margin-left:0px;
height:auto;
overflow:auto;
position:absolute;
width:183px;
border: 1px #B7D9ED solid;
border-top:1px transparent solid;
list-style-type:none;
display:none;
}
/*li*/
.ListPart .ComboListItem
{
display:block;
width:inherit;
height:21px;
padding: 1px 0px 0px 3px;
}
/*when mouse move */
.ComboListItem:hover
{
background-image:url('images/BGline.png');
background-repeat:repeat-x;
}
Java script aspects:
for js point of view we just consider the operation,methods and properties that the combolist have to contain, for example the selected index ,
selected item , add new item,remove item ,and high level operations like sorting of item.

Code
//style nice looking when mouse move,ou,up
/
function changeState( element ,height ,statNumber)
{
//statNumber the number of state we want to show currently
// elemnt is imageBackground for span
/// 0: normal status,1 mouseMove status,2 mousepress status, 3 fucuse,4 disable ,5 no
var top=height*statNumber;
// alert('100% -'+left+'px');
document.getElementById(element).style.backgroundPosition='100% -'+top+'px';
}
function chageBorderColorWhenMouseMove(element)
{
document.getElementById(element).style.borderBottom='1px #3D7BAD solid';
document.getElementById(element).style.borderRight='1px #3D7BAD solid';
document.getElementById(element).style.borderLeft='1px #3D7BAD solid';
}
function chageBorderColorWhenMouseOut(element)
{
document.getElementById(element).style.borderBottom='1px #B7D9ED solid';
document.getElementById(element).style.borderRight='1px #B7D9ED solid';
document.getElementById(element).style.borderLeft='1px #B7D9ED solid';
}
function ShowComboList(element)
{
document.getElementById(element).style.display='block';
}
function HideComboList(element)
{
document.getElementById(element).style.display='none';
}
//----------------------selection and add new items
var ComboListItemIndex=-1;
var ComboListSelectedindex=-1;
var ComboListselectedItem="";
function ComboListAddNewListItem(ComboxListId)
{
ComboListItemIndex=ComboListItemIndex+1;
var NewItemString="<li id=ComboListItem"+ComboListItemIndex+" class=ComboListItem onclick=ComboListSelectedIndex(this);ComboListSelectedItem(this);>item"+ComboListItemIndex+"</li>";
var listBoxOldElemtns=document.getElementById(ComboxListId).innerHTML;
var newListboxItem=listBoxOldElemtns+NewItemString;
document.getElementById(ComboxListId).innerHTML=newListboxItem;
}
// return the selected index of the combo list
function ComboListSelectedIndex(elThis)
{
var name=elThis.id;
// get the index.
ComboListSelectedindex=name.charAt((name.length)-1);
return ComboListSelectedindex;
}
function ComboListSelectedItem(elThis)
{
var Item=elThis.innerHTML;
document.getElementById('InputBody').value=Item;
HideComboList('ListPart');
return Item;
}
//
function ComboListGetItem(index)
{
var re="";
if(index>-1 && index<=ComboListItemIndex)
{
var name="ComboListItem"+index;
re=document.getElementById(name).innerHTML;
}
else
{
alert('Out Of Rang Index');
}
return re;
}
// SET VALUE IN SPECIFIC INDEX POSITION
function ComboListSetItem(index,value)
{
if(index>-1 && index<=ComboListItemIndex)
{
var name="ComboListItem"+index;
document.getElementById(name).innerHTML=value;
}
else
{
alert('Out Of Rang Index');
}
}
// FUNCTION LENGTH;
//-1 for empty.
function ComboListLength()
{
return ComboListItemIndex;
}
sorting element of combolist :
whatever you used mean the sorting algorithm ,insertion sort,quicksort ,heapsort ,just invoke the combolist sort method like code below ,
here i used the array bufferCombolist ,js provide sorting function for array
function ComboListSort()
{
ComboListBuffer.sort();
for(var x=0;x<=ComboListLength();x++)
{
ComboListSetItem(x,ComboListBuffer[x]);
}
}
html aspect :
from html point of view we just think the combolist as below:
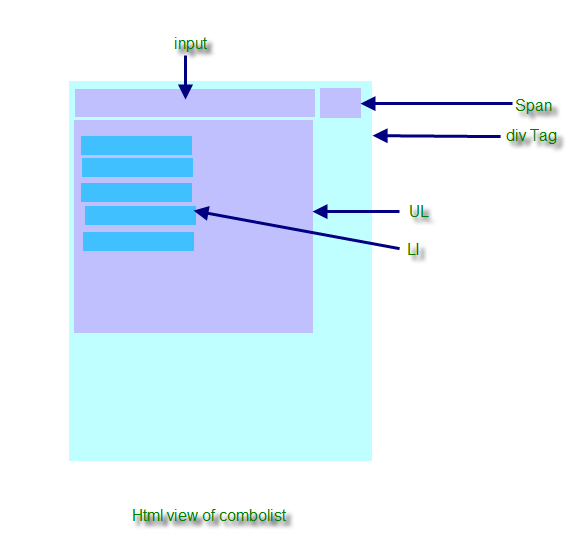

Code
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta content="text/html; charset=utf-8" http-equiv="Content-Type" />
<title>Untitled 1</title>
<link rel="stylesheet" type="text/css" href="combolist.css"/>
<script language="javascript" type="text/javascript" src="combolist.js"></script>
</head>
<body>
<div class="ComboList">
<span id="HeaderSpan" class="headPar" onmousemove="changeState('HeaderSpan',21,1);chageBorderColorWhenMouseMove('InputBody');" onmouseout="changeState('HeaderSpan',21,0);chageBorderColorWhenMouseOut('InputBody')" onmousedown="changeState('HeaderSpan',21,2);ShowComboList('ListPart');" onmouseup="changeState('HeaderSpan',21,1);"></span>
<input id="InputBody" class="bodyPart" value=""/>
<ul id="ListPart" class="ListPart">
</ul>
</div>
<a href="#" onclick="ComboListAddNewListItem('ListPart');">add New Item </a>
<a href="#" onclick="ComboListGetItem('2');">get(i) </a>
<a href="#" onclick="ComboListSetItem('2','AMMAR');">set(i,value)</a>
</body>
</html>
Tag标签: css,js,web,combolist